Introduction
Eslint Git Hook is a repository for scripts that run automatically and detects and In recent years, many applications have been created using javascript, typescript, and other frameworks. The code and logical approach are excellent for solving many complex problems. However, when it comes to coding standards, not many developers use lint. We’re about to solve this issue by combining ESLint and Git Pre-commit. So let’s talk about ESLint’s features and implementation here.
ESLint
The ESLint tool generally analyses source code and helps to identify bugs, syntactical errors, and many more. Hence gives a way to fix those bugs in an easy way by providing more hints in a human-readable format which makes work simpler. Now let’s have a look at the following example scenario of ESLint.
Example Scenario
For an instance have a look at the following example

For an instance have a look at the following example.
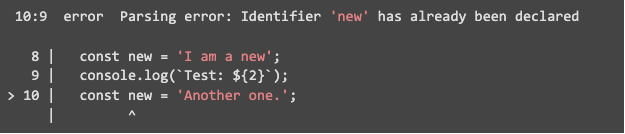
Use Case
- To provide consistent code style
- Own linting rules can be added
- Keeping track of errors dynamically
- Bugs are flagged during syntax errors
- Suggestions for best practices are notified
Implementing ESLint in IDE
Most developers implement ESLint to IDE as an extension. If we are using Visual Studio as an IDE it is available as an extension that can be downloaded as shown.
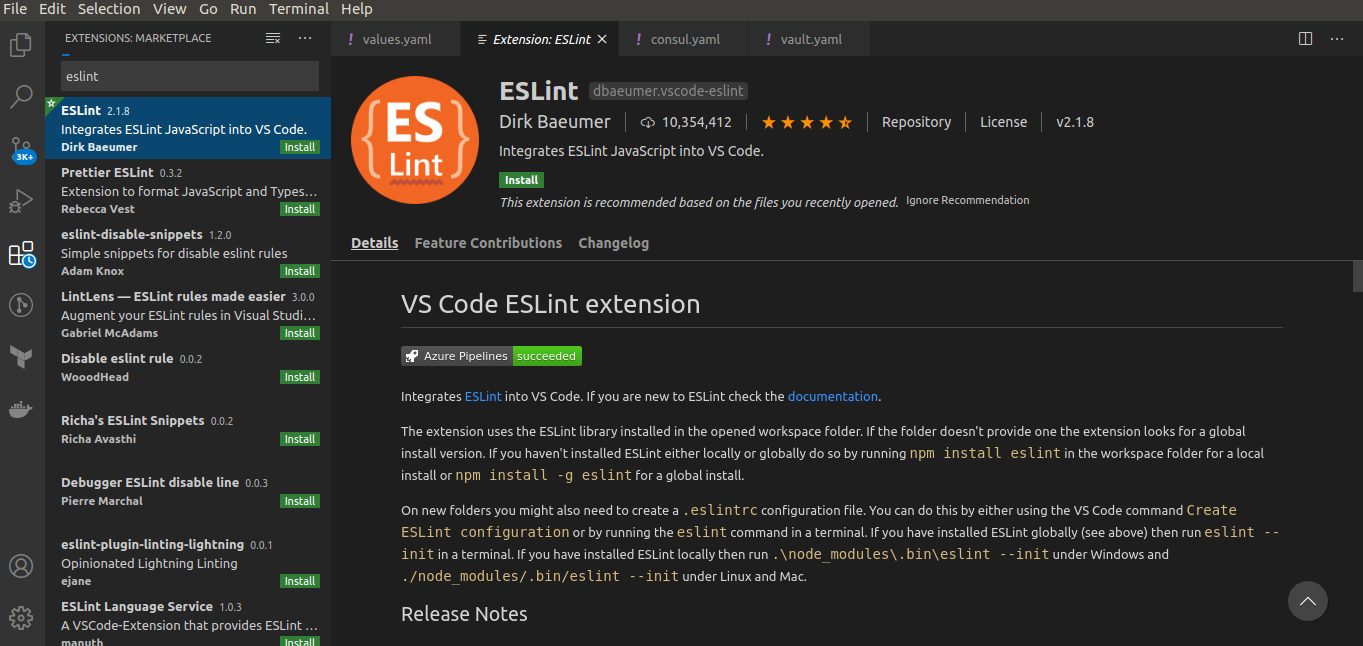
Installing ESLint
The ESLint can be installed globally by the following command in the terminal npm install -g eslint This command installs eslint globally in our machine. If we want to install in project-specific folder, the commands may slightly differ they are available in the official ESLint Documentation.
Now it’s installed, we need to configure it. The config file will allow us define which features and rules we want to use. if we want our linter to recognise ES6 syntax but break when an undefined variable is present,we need a config file. To make one, run eslint -init as shown below.

In my case I select to check syntax and styling
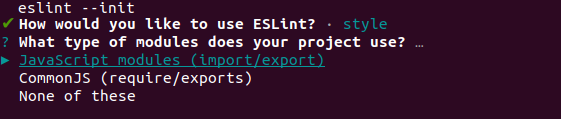
Since, we are using import and export as modules in our code,here I have selected JavaScript modules(import/export
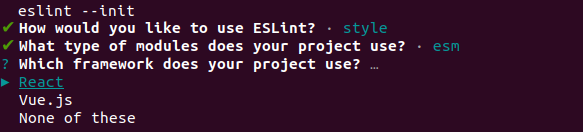
As Iam using React, I have selected react as framework.


It asks about popular style guide and the default are with codes at their respective Github repo as follows:
Finally, it asks the configuration file format, which can be JSON, YAML, or JavaScript.
This will create a file in our root called eslintrc.json with default rules such as debugger which we can change later.
Now default setup is done. To get configuration suggestions or to start linting files with the command eslint**/*.js and it shows issues and warnings in our code. The most recommended command is ./node_modules/.bin/eslint src which on execution gives the output as below
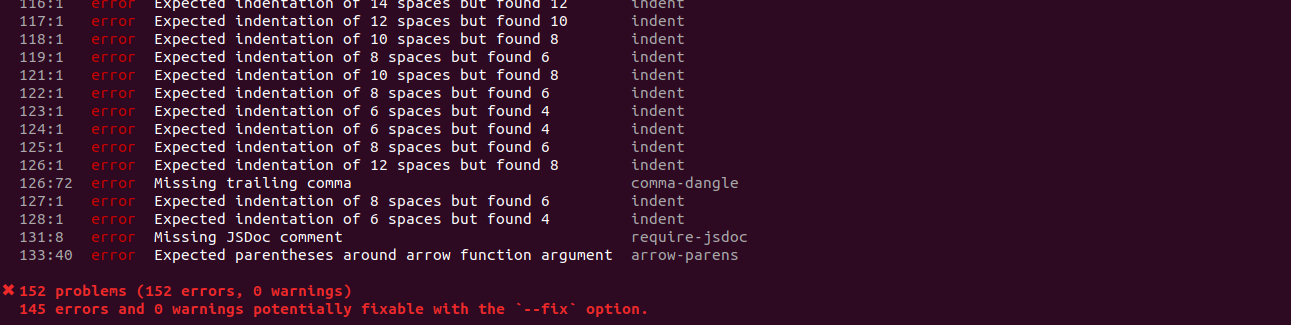
Custom Formatters
ESLint supports the creation of custom formatters. Custom formatters can be created directly in our project or as a npm package to be distributed separately.
Each formatter is just a function that receives a result object and returns a string. In the following example json build-in formateer is implemented.
//my-demo-formatter.js
module.exports = function(results) {
return JSON.stringify(results, null, 2);
};
You can use the -f (or -formatter) command line flag to run ESLint with this formatter:
eslint -f ./my-demo-formatter.js src/
Packaging Custom Formatters
To create a formatter as npm package create a package with name in the format of eslint-formatter-* , Here * is the name of the formatter (such as eslink-formatter-demo).In projects we can use the formatter with -f (or –formatter) flag like shown below
eslint -f demo src/
Now ESLint set up for our project is achieved through simple steps.
If the developer couldn’t fix the lint issue due to some reasons, we can overcome this as git provides a way of pre-commit. We are yet to discuss git pre-commit. Before diving in we may need a mild knowledge of git hooks
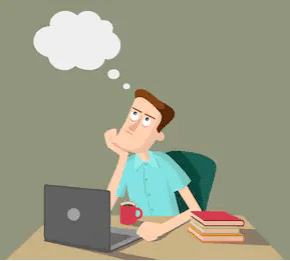
Hooks in Git
- Git provides a way to execute custom scripts during certain important actions. It has two groups of these hooks client-side and server-side.
- The client-side hooks are triggered during operations such as committing and merging.
- Server-side hooks are triggered during operations such as receiving pushed commits over the network.
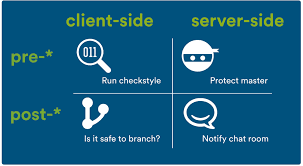
In general. In all git repositories, hooks are enabled by default.
$ cd .git/hooks/me);
In general .git has default hooks in all git repositories.

Each file has some unique works. For example if we execute git commit, pre-commit hook will execute. Here now we are focusing on pre-commit for ESLint.
Pre-Commit
The pre-commit hook runs first, before you even type in a commit message. It’s used to inspect the snapshot that’s about to be committed, to see if you’ve forgotten anything, to ensure tests run, or to examine whatever you need to inspect in the code..
For developers to commit the code, a pre-commit is run to check all available lint configurations here and abort the commit if any lint issues are found. It is done using a script file. The bash removes the default pre-commit file and creates a new file with lint error checks enabled. The bash script is here as shown below:
#!/bin/bash
cd .git/hooks
FILE=pre-commit.sample
if [[ -f “$FILE” ]]; then
rm -rf pre-commit.sample
fi
touch pre-commit
cat >pre-commit <<EOF
#!/bin/bash
\$(cd ..)
\$(cd ..)
LINT_PROC=\$(./node_modules/.bin/eslint src)
echo “<<—– Checking the lint issue —–>>”
echo “\$LINT_PROC”
if [ “\$LINT_PROC” == “” ]; then
echo “<<—– No lint is found, u may commit code —–>>”
exit 0
fi
echo “<<—– Since lot of lint issue is found, plz fix the above issue and then commit —–>>”
exit 1
EOF
chmod +x pre-commit
Here the above script file is placed in the project root folder.Once cloned the project as a first thing, we must execute the script or we may be unable to use the git pre-commit.
The above script executes the lint package to find out the lint issue. If any lint issue is detected we are unable to commit the code.
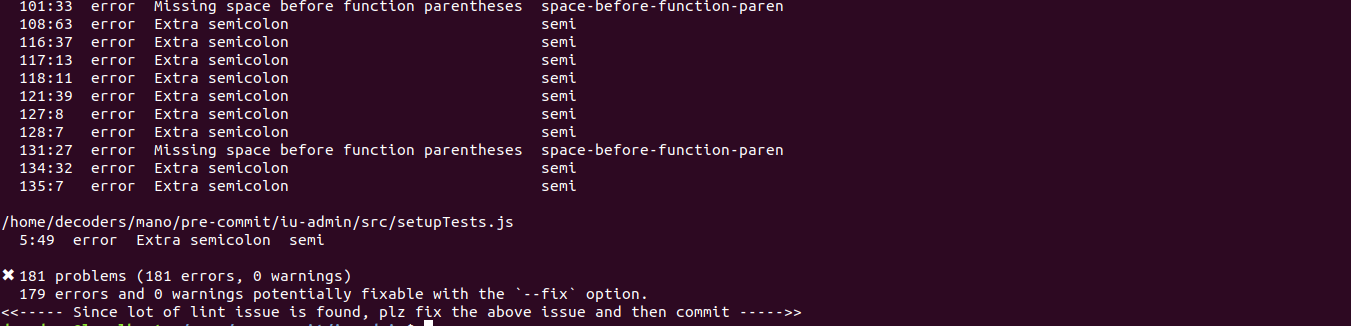
Thus pre-commit triggers on git commit and check for lint issues. Once you fix the errors we are happy to commit the code.
Conclusion
We have implemented the ESLint in our project and also done with pre-commit that led our teams to improve the code quality and style.